Your cart is currently empty!
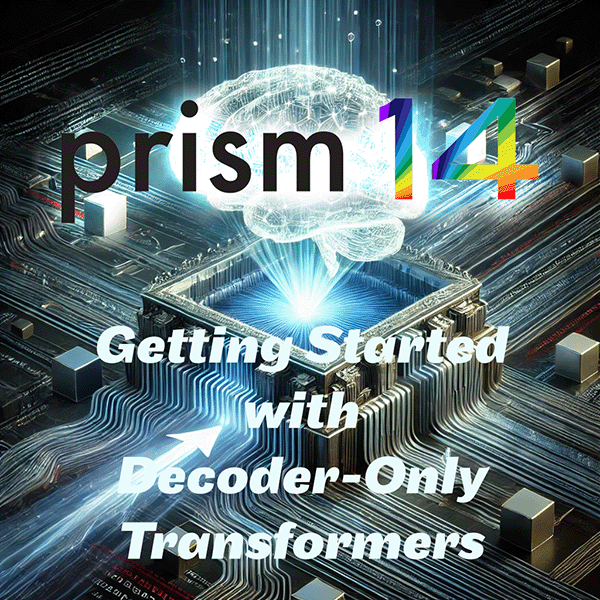
How to Get Started with Decoder-Only Transformers
Get started with decoder-only transformers, like OpenAI’s GPT models! Decoder-only transformers have gained massive popularity due to their success in tasks like text generation, summarization, dialogue systems, and code generation. These models utilize only the decoder portion of the original transformer architecture, focusing on generating sequences autoregressively—meaning they predict the next token in a sequence based on the previously generated tokens. If you’re interested in working with these models, here’s a step-by-step guide to get you started:
1. Understand the Architecture
Before diving into code, it’s crucial to understand how decoder-only transformers work to get started with decoder-only transformers. Here’s a simplified overview:
- Architecture: In a decoder-only transformer, there is no separate encoder. The model takes an input sequence and predicts the next token one step at a time.
- Autoregressive Task: Unlike encoder-decoder transformers (e.g., for translation), decoder-only transformers predict each word based on the previous tokens, making them ideal for text generation tasks.
- Self-Attention: The model uses self-attention to determine which tokens in the sequence are relevant to the current token generation, considering past tokens but not future ones.
2. Choose Your Framework
There are several libraries and frameworks available for implementing and fine-tuning decoder-only transformers — this is the second big step to get started with decoder-only transformers:
- Hugging Face’s Transformers Library: Hugging Face is an industry-standard for working with pre-trained models like GPT-2 and GPT-3. It’s a great starting point because it allows you to leverage existing pre-trained models, fine-tune them on custom data, and deploy them with ease.
- PyTorch: For lower-level understanding and customization, PyTorch is a great library to build transformers from scratch or modify existing architectures.
- TensorFlow: TensorFlow also provides tools for implementing transformers, though it tends to be more popular for encoder-decoder architectures.
3. Start with Pre-trained Models
The fastest way to get started with decoder-only transformers is to use pre-trained models available through Hugging Face’s model hub. Here’s how you can use GPT-2 or GPT-3 from Hugging Face:
pip install transformers
Then, in Python:
from transformers import GPT2LMHeadModel, GPT2Tokenizer
# Load pre-trained model and tokenizer
model = GPT2LMHeadModel.from_pretrained("gpt2")
tokenizer = GPT2Tokenizer.from_pretrained("gpt2")
# Encode input text
input_text = "The future of AI is"
input_ids = tokenizer.encode(input_text, return_tensors='pt')
# Generate text
output = model.generate(input_ids, max_length=50, num_return_sequences=1)
# Decode the output
generated_text = tokenizer.decode(output[0], skip_special_tokens=True)
print(generated_text)
4. Fine-Tuning a Decoder-Only Model
If you want to customize a pre-trained model for a specific task (e.g., generating domain-specific text), you can fine-tune a decoder-only transformer on your own dataset.
Steps for Fine-Tuning:
- Prepare Dataset: Create a dataset formatted as input-output pairs, where you provide the model with a prompt and its correct response.
- Load Dataset into Hugging Face:
from datasets import load_dataset dataset = load_dataset('your_dataset_here')
- Fine-Tune the Model:
from transformers import Trainer, TrainingArguments training_args = TrainingArguments( output_dir="./results", num_train_epochs=3, per_device_train_batch_size=4, per_device_eval_batch_size=4, warmup_steps=500, weight_decay=0.01, logging_dir="./logs", ) trainer = Trainer( model=model, args=training_args, train_dataset=dataset["train"], eval_dataset=dataset["validation"], ) trainer.train()
This fine-tunes the model based on your dataset and training arguments. Fine-tuning allows you to adapt the model for specific tasks like generating text in a particular domain, improving accuracy for that use case.
5. Train from Scratch (Optional)
If you’re interested in building a decoder-only transformer from scratch, this requires a deeper understanding of the transformer architecture. You’ll need to:
- Implement self-attention: Key, query, and value matrices must be generated for every token, with attention weights computed and normalized.
- Implement masking: Since the model should not attend to future tokens, you’ll need to apply a mask to prevent the model from looking ahead.
- Train on large-scale data: These models require a significant amount of data and computational resources to train effectively.
There are resources, such as Andrej Karpathy’s miniGPT, that can guide you through building a transformer-like architecture from scratch using PyTorch.
6. Experiment with Generation Settings
Once you have a working model, you can tweak its performance by adjusting the generation settings:
- Temperature: Controls the randomness of predictions. A higher temperature produces more random outputs, while lower temperatures result in more conservative outputs.
- Max Length: Limits the number of tokens the model will generate.
- Top-k Sampling: Restricts the model to choosing from only the top-k most likely tokens during generation.
- Top-p (Nucleus) Sampling: Limits the model to sampling from tokens whose cumulative probability adds up to a specified value (e.g., 0.9).
Example:
output = model.generate(input_ids, max_length=100, num_return_sequences=1, temperature=0.7, top_k=50, top_p=0.9)
7. Evaluate and Optimize
- Perplexity: This metric can help you evaluate how well your model is performing in generating coherent sequences.
- Manual Evaluation: Since text generation is subjective, it’s important to manually inspect the output of your model to ensure it meets your expectations.
Resources to Dive Deeper:
- Hugging Face Course: Learn more about transformers and fine-tuning with practical examples Hugging Face Course.
- Andrej Karpathy’s miniGPT: Build a transformer model from scratch with PyTorch GitHub repo.
- Transformer Architecture Paper: The original paper by Vaswani et al., Attention Is All You Need, gives you foundational knowledge on transformers.
By following these steps, you’ll be well on your way to mastering decoder-only transformers and applying them to various tasks like text generation, dialogue systems, or code completion!
More on Machine Learning and AI Fundamentals at Prism14
To get occasional updates from Prism14 and info directly in your inbox ==>
==> Subscribe to Prism14’s Update
Book an Appointment ==> Book Now to Learn or Integrate With Prism14
by
Leave a Reply